I like to use generative AI to brainstorm solutions. It is a great way to learn something new.
While I mainly use ChatGPT in my daily work, I like to keep up to date with the major alternatives. So, in my latest task, I used the same initial prompt with these tools:
All examples were generated on August 31st, 2023.
Goals
The Tracking add-on for Advanced Ads comes with a function to generate dummy impression and click data for testing. The previous version generated unrealistic data with too high CTRs and little differences between ads.
My goal was to lower the CTR to circle around 3% and add a tendency for some ads to have a generally lower CTR than others.
I wrote the following prompt and asked all three generative AI tools for suggestions before writing the actual code.
I gave it the whole function code in my prompt, but left out the irrelevant parts below.
Initial Prompt
The following function generates dummy tracking data for a plugin. I want to change it to make it more “natural”.
One change would be to have the values for $values_clicks to be around 3% of the values for $values. Currently, it seems to go up to 30%.
I also want individual ids to have a tendency. E.g., some ids have rather lower numbers than others. If the function runs again, this tendency should be similar. Please don’t write any code.
Just give me an outline of the changes you would suggest with specific reference to the line in the code or parts of the code you want to change and how.
…
foreach ( $sub_ids as $sub_id ) {
$sub_id = $ids[ $sub_id ];
// Create random data.
array_push( $values, $ts, $sub_id, ( ( wp_rand( 0, 10 ) + wp_rand( 0, 10 ) ) * wp_rand( 1, 5 ) ) );
array_push( $values_clicks, $ts, $sub_id, ( ( wp_rand( 0, 2 ) + wp_rand( 0, 3 ) ) * wp_rand( 1, 3 ) ) );
…
}
…
Code language: PHP (php)
ChatGPT
First reply
Making $values_clicks
Around 3% of $values
- Current Code:
array_push( $values_clicks, $ts, $sub_id, ( ( wp_rand( 0, 2 ) + wp_rand( 0, 3 ) ) * wp_rand( 1, 3 ) ) );
- Suggested Change: Instead of generating a random number for
$values_clicks
, calculate it as approximately 3% of the corresponding value in$values
. You can still add a small random factor to make it look natural.
- Suggested Change: Instead of generating a random number for
Adding Tendency for Individual IDs
- Initialization: Add a new associative array to store the tendency for each
ad_id
. Initialize it outside the$runs
loop. - Inside the Loop for
$sub_ids
:- Current Code:
array_push( $values, $ts, $sub_id, ( ( wp_rand( 0, 10 ) + wp_rand( 0, 10 ) ) * wp_rand( 1, 5 ) ) );
- Suggested Change: Use the tendency value for the
ad_id
from the associative array to influence this random number generation. Update the tendency value for thead_id
in the associative array based on the newly generated value.
- Current Code:
My take on this
Good suggestion on the first problem to calculate clicks based on the impressions and not individually. It’s even better that ChatGPT suggested adding a random factor on top of that. This aligns perfectly with my goal.
The second suggestion doesn’t seem to understand my problem of keeping the tendency persistent. I could have been more clear on this.
Second reply
My prompt
Let’s proceed with the first problem. How would that changed line of code look like?
ChatGPT
Certainly! To make $values_clicks
around 3% of $values
, you can modify the line where $values_clicks
is populated. You can use the value that you generated for $values
and take approximately 3% of it, perhaps adding a small random factor for natural variation.
Here’s how the changed line of code might look:
// Assuming $value is the generated value for $values
$value = ( ( wp_rand( 0, 10 ) + wp_rand( 0, 10 ) ) * wp_rand( 1, 5 ) );
// Generate $value_click as approximately 3% of $value
$value_click = round($value * 0.03 * (wp_rand( 95, 105 ) / 100));
// Populate $values and $values_clicks
array_push( $values, $ts, $sub_id, $value );
array_push( $values_clicks, $ts, $sub_id, $value_click );
Code language: PHP (php)
In this example, $value_click
is calculated as 3% of $value
. The term (wp_rand( 95, 105 ) / 100)
adds a random factor to make it vary between 2.85% to 3.15% of $value
, making the data look more natural.
Remember to replace this logic in the appropriate place in your existing code.
My take on this
I am very happy with this suggestion. However, I adjusted the variation a bit, fixed the code style, and removed single-use variables.
Third reply
Let’s go to the second problem now with my next prompt. Looking back, I think I gave the possible solution away with my last sentence.
My prompt
Now about the next problem. Your suggestion missed that I want the tendency to be consistent, also when running the code a second time. I don’t see that in your suggestion. I don’t want to store it in the WP options either. Can I generate a static value out of something else, e.g., the id?
ChatGPT
Ah, I see what you’re aiming for. If you want the tendency to be consistent across multiple runs without storing it in the database, you can indeed generate a static value based on the ad_id
. One way to do this is to use a hash function that takes the ad_id
as input and returns a value that you can use to influence the random number generation for that ad_id
.
Here’s a conceptual outline:
- Hash Function: Create a simple hash function that takes an
ad_id
and returns a value between a specific range, say 0.5 to 1.5. This value will serve as the tendency multiplier for thatad_id
. - Inside the Loop for
$sub_ids
:
- Current Code:
array_push( $values, $ts, $sub_id, ( ( wp_rand( 0, 10 ) + wp_rand( 0, 10 ) ) * wp_rand( 1, 5 ) ) );
- Suggested Change: Use the hash function to get the tendency multiplier for the current
ad_id
. Multiply the random value by this multiplier.
Here’s a sample hash function and how you might use it:
// Hash function to generate a tendency multiplier for an ad_id
function get_tendency_multiplier($ad_id) {
return 1 + (crc32($ad_id) % 100) / 100; // This will return a value between 1 and 1.99
}
// Inside your loop for $sub_ids
$tendency_multiplier = get_tendency_multiplier($sub_id);
array_push( $values, $ts, $sub_id, round(( ( wp_rand( 0, 10 ) + wp_rand( 0, 10 ) ) * wp_rand( 1, 5 ) ) * $tendency_multiplier) );
Code language: PHP (php)
This way, the tendency for each ad_id
will be consistent across multiple runs of the code, as it’s generated based on the ad_id
itself.
My take on this
Again, great solution to build up on this after some changes. It definitely saved me some research time about hash functions, which I could then invest in learning more about crc32()
.
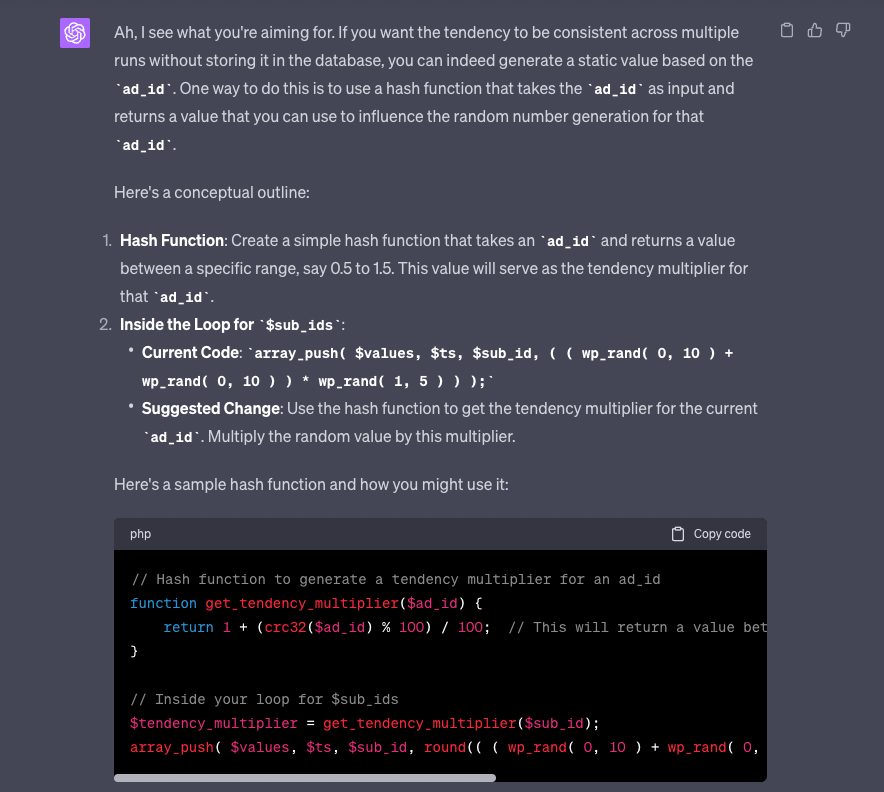
Claude
First reply
Here are some suggestions to make the data more natural in the create_test_data() function:
- For $values_clicks, instead of having the values randomly between 0-5, you could make it a percentage of the $values count, like 3-5%. This would link the clicks to the impressions in a more realistic way.
- To make some IDs have consistently higher/lower values, you could create a bias array before the loop, with each ID mapped to a modifier number (e.g. 0.5 to 1.5). Then in the loop you can multiply the random value by the modifier for that ID.
- To make the tendencies persist between runs, you can save the bias array in a class property or external cache and reuse it.
- To introduce some randomness, you can occasionally shuffle the bias array, or slowly change the modifiers over time.
- You may want to make the day of week affect the values also, e.g. increase on weekdays, lower on weekends.
- Rather than completely random days, it may look more natural if some dates are closer together in clusters, related to hypothesized campaign or seasonal trends.
So in summary, the key changes would be:
- Relate clicks to impressions
- Introduce ID biases that persist between runs
- Add some real-world variables like day of week seasonality
- Make the dates cluster together more realistically
Let me know if any part needs more clarification!
Here are some suggestions to make the data more natural in the create_test_data() function:
- For $values_clicks, instead of having the values randomly between 0-5, you could make it a percentage of the $values count, like 3-5%. This would link the clicks to the impressions in a more realistic way.
- To make some IDs have consistently higher/lower values, you could create a bias array before the loop, with each ID mapped to a modifier number (e.g. 0.5 to 1.5). Then in the loop you can multiply the random value by the modifier for that ID.
- To make the tendencies persist between runs, you can save the bias array in a class property or external cache and reuse it.
- To introduce some randomness, you can occasionally shuffle the bias array, or slowly change the modifiers over time.
- You may want to make the day of week affect the values also, e.g. increase on weekdays, lower on weekends.
- Rather than completely random days, it may look more natural if some dates are closer together in clusters, related to hypothesized campaign or seasonal trends.
So in summary, the key changes would be:
- Relate clicks to impressions
- Introduce ID biases that persist between runs
- Add some real-world variables like day of week seasonality
- Make the dates cluster together more realistically
Let me know if any part needs more clarification!
My take on this
It solved the first question with a variation as well, perfect.
I really liked the approach to the second problem. Since I didn’t mention how I wanted to keep the tendency, storing it in some persistent cache made sense. I also like the suggestions about using the date of the week, though I am not going to use it.
Second try
My prompt
Thanks. Let’s proceed with point 1. How would the code for this look like?
Claude
Here is how you could update the code to make the $values_clicks be a percentage of the $values counts:
// Create random data
$impression_count = wp_rand(10, 50);
array_push($values, $ts, $sub_id, $impression_count);
$click_percentage = wp_rand(3, 5) / 100;
$click_count = round($impression_count * $click_percentage);
array_push($values_clicks, $ts, $sub_id, $click_count);
Code language: PHP (php)
The key changes:
- Generate a random impression count first and store it
- Calculate a random click percentage between 3-5%
- Multiply the impressions by the percentage to get clicks
- Push the clicks to the separate array
This makes the clicks dependent on the impressions, and keeps the percentage within the desired range.
Let me know if you would like me to explain any other points further!
My take on this
While the solution is viable with a bit of cleanup, I didn’t like that it invented a new algorithm to calculate impressions (first line) without telling me so.
In this particular case, the original code is not mine, and I don’t see a reason to change it.
When I asked Claude about why it made this change, it told me that it found that the original algorithm created too large differences between impressions between ads. I don’t consider this a problem here, though.
Third reply
Let’s go over to the next prompt addressing the second goal.
My prompt
Now about the next problem. Your suggestion missed that I want the tendency to be consistent, also when running the code a second time. I don’t see that in your suggestion. I don’t want to store it in the WP options either. Can I generate a static value out of something else, e.g., the id?
Claude
You’re right, my previous suggestion didn’t fully address creating persistent tendencies for each ID across multiple function calls.
Instead of storing the biases externally, we can generate them procedurally based on the ID itself.
Here is one way to do it:
- Create a bias generation function that takes the ID and returns a consistent modifier value each time:
function getIdBias($id) {
// Hash the ID to get a consistent pseudo-random int
$hash = hash('crc32', $id);
// Map hash to range 0.5 - 1.5
$bias = (hexdec($hash) / 0xFFFFFFFF) + 0.5;
return $bias;
}
Code language: PHP (php)
- When generating the impression count, multiply it by the bias for that ID:
php $impressionCount = wp_rand(10, 50) * getIdBias($id);
- The bias will be the same each time for a given ID, but different across IDs.
This creates a persistent tendency for each ID without storing anything externally. Let me know if this makes sense or if you need any clarification!
My take on this
The solution is similar to the one ChatGPT gave and one to build upon and learn from.
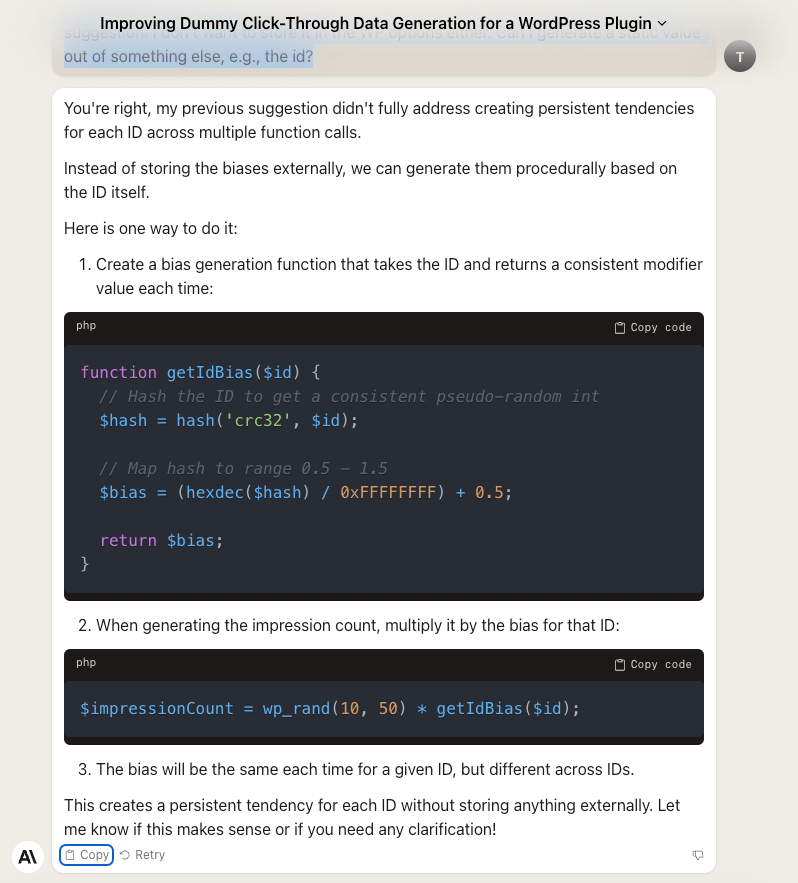
Bard
First reply
Sure, here are the changes I would suggest:
- To make the values for $values_clicks be around 3% of the values for $values, you can change the following line of code:
PHP
array_push( $values_clicks, $ts, $sub_id, ( ( wp_rand( 0, 2 ) + wp_rand( 0, 3 ) ) * wp_rand( 1, 3 ) ) );
Code language: PHP (php)
to:
PHP
array_push( $values_clicks, $ts, $sub_id, ( ( wp_rand( 0, 2 ) + wp_rand( 0, 3 ) ) * wp_rand( 1, 3 ) ) * 0.03 );
Code language: PHP (php)
- To make individual ids have a tendency, you can use the following code:
PHP
// Create a random tendency for each ad id.
$tendencies = [];
foreach ( $ids as $id ) {
$tendency = wp_rand( -10, 10 );
$tendencies[ $id ] = $tendency;
}
// Apply the tendency to the data.
foreach ( $values as &$value ) {
$value[2] += $value[2] * $tendencies[ $value[1] ];
}
foreach ( $values_clicks as &$value ) {
$value[2] += $value[2] * $tendencies[ $value[1] ];
}
Code language: PHP (php)
This code will create a random tendency for each ad id, and then apply that tendency to the data. This will make it more likely that some ids will have lower numbers than others, and that this tendency will be consistent from run to run.
I hope this helps!
My take on this
What about my instruction of “Please don’t write any code.”?
It solved the first problem by calculating 3% of the calculated clicks, though I instructed it to base it on the $values
. Reducing one factor in the clicks calculation would have been a viable solution, but it was already lower than $values
so a higher factor would have done it. Bard was so far off with the first task that I didn’t iterate on it.
As expected, the second goal is also not resolved. So let’s give it a second change with that.
Second try
My prompt
Now about the next problem. Your suggestion missed that I want the tendency to be consistent, also when running the code a second time. I don’t see that in your suggestion. I don’t want to store it in the WP options either. Can I generate a static value out of something else, e.g., the id?
Bard
Yes, you can generate a static value out of the ad id. Here is one way to do it:
PHP
// Create a static value for each ad id.
$tendencies = [
1 => 1,
2 => 2,
3 => 3,
4 => 4,
5 => 5,
];
// Apply the tendency to the data.
foreach ( $values as &$value ) {
$value[2] += $value[2] * $tendencies[ $value[1] ];
}
foreach ( $values_clicks as &$value ) {
$value[2] += $value[2] * $tendencies[ $value[1] ];
}
Code language: PHP (php)
This code will create a static value for each ad id, and then apply that value to the data. This will make the tendency consistent from run to run, even if the code is run multiple times.
Another way to do it is to use the following code:
PHP
// Create a random tendency for each ad id, but store it in the database.
$tendencies = [];
foreach ( $ids as $id ) {
$tendency = wp_rand( -10, 10 );
$this->wpdb->insert(
$this->tendencies_table,
[
'ad_id' => $id,
'tendency' => $tendency,
]
);
$tendencies[ $id ] = $tendency;
}
// Apply the tendency to the data.
foreach ( $values as &$value ) {
$value[2] += $value[2] * $tendencies[ $value[1] ];
}
foreach ( $values_clicks as &$value ) {
$value[2] += $value[2] * $tendencies[ $value[1] ];
}
Code language: PHP (php)
This code will create a random tendency for each ad id, but store it in the database. This will make the tendency consistent from run to run, even if the code is run multiple times. However, it will also require you to create a new table in the database.
Which method you choose will depend on your specific needs.
My take on this
While the first suggestion is to create a static array with static tendencies, the second one uses some non-existing pseudo-WordPress functions. I didn’t even bother to proceed with Bard after this.
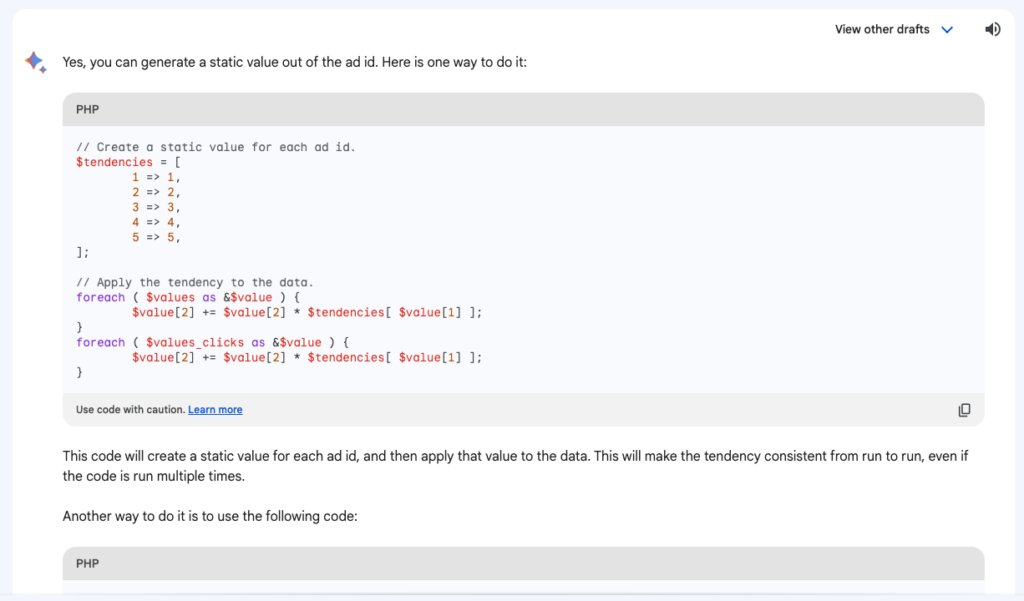
Conclusions
I made similar comparisons in the past, with ChatGPT always coming out on top of the contestants. This time, Claude was a suitable contestant. Not bad for a generative AI that doesn’t charge yet.
I know that some marketing people prefer Google Bard, but personally, I haven’t found a use case in which it gave me better results than any of the other tools.
What this test confirmed again was that these tools are great virtual assistants that can help brainstorm alternative solutions, but they are not suitable for using the generated code without understanding every line.
I personally like asking follow-up questions to learn about their reasoning since it helps me learn.